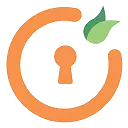
WordPress REST API Authentication plugin provides the security from unauthorized access to your WordPress REST APIs.
Search Results :
×OAuth 2.0 is the most opted method for authenticating access to the APIs. OAuth 2.0 allows authorization without the need providing user's email address or password to external application. This method of WordPress REST API OAuth 2.0 Authentication involves the use of OAuth 2.0 protocol flow to obtain the security access token or id token (JWT token) and that token will be used to authenticate the WordPress REST API endpoints. Each time a request to access the WordPress REST API endpoint will be made, the authentication will be done against that access token/id token (JWT token), and on the basis of the verification of that API Bearer token, the resources for that API request will be allowed to access.
The token provided using the OAuth 2.0 Authentication Method is highly encrypted and secure, hence security is not compromised. It is the most secure method to authenticate/ protect WordPress REST API endpoints. This method is somewhat similar to the JWT authentication method but far more secure due to various benefits of OAuth 2.0 protocol.
WordPress REST API Authentication plugin provides the security from unauthorized access to your WordPress REST APIs.
Use Case: Register WordPress user or Authenticate /login users in external platforms based on WordPress credentials via WordPress REST API
Suppose you have a login form in your application and want to authenticate
the user on the basis of their WordPress login credentials, then OAuth 2.0
Authentication in REST API method will help you in achieving that. The
plugin provides a WordPress login API. You can easily pass the WordPress
credentials of that user to this API and based on the validation, you will
receive the authentication and response.
Also, Implementing OAuth 2.0 Authentication in REST API method can also be
used to register users in WordPress using the admin credentials of
WordPress user. Using the plugin OAuth 2.0 token endpoint, you can pass
WordPress user credentials which have administrator capabilities such that
token generated will have admin capabilties can be used to perform
operations like user registration for which administrative privileges are
required. Once you have the token, you can use this token with wordPress
‘/users’ API to register users in WordPress via REST API request.
Use Case: Authenticate/ protect WordPress REST API Endpoints securely or register users in WordPress without using admin user credentials.
If you want to access the WordPress REST API without passing the WordPress
user credentials or want to register the users in WordPress securely
without need to pass the admin user credentials of WordPress and instead
pass the client credentials provided by the plugin, then this method is
the perfect solution such that there won’t be any chance of user
credentials getting compromised.
The plugin acts both as a OAuth 2.0 Identity provider(Server) which
provides the token and REST APIs authenticator for authentication of these
WordPress REST API endpoints on the basis of the token. Hence it provides
utmost security to obtain the token and that token can be used to
authenticate the REST API request.
1. The REST API request will be made with appropriate parameters to obtain the token for our plugin. Our plugin will act as an OAuth 2.0 Identity provider and provides the access token.
2. The actual REST API request to access the resource will be made with the access token received from the last step passed in the Authorization header with token type as Bearer. The plugin now acts as Authenticator to authenticate the API on the basis of token validity. If the token is validated successfully then the API requester will be allowed to access the resource else on the failed validation the error response will be returned.
Related Usecase:
Now the plugin setup part has been done successfully. Below comes the part in which the actual security access/JWT token will be obtained and used further for accessing the WordPress REST APIs.
Request: POST https://<domain-name>/wp-json/api/v1/token
Body:
grant_type =<password>
username =<wordpress username>
password = <wordpress password>
client_id =<client id>
Sample curl Request Format-
curl -d "grant_type=password&username=<wordpress_username>&password=<wordpress_password>&client_id=<client_id>"
-X POST http://<wp_base_url>/wp-json/api/v1/token
-H 'app-name:TheAppName'
Request: POST https://<domain-name>/wp-json/api/v1/token
Body:
grant_type = <refresh_token>
refresh_token = <Refresh Token>
Sample curl Request Format-
curl -d "grant_type=refresh_token&refresh_token=<refresh_token>&client_id=<client_id>&client_secret=<client_secret>"
-X POST http://<wp_base_url>/wp-json/api/v1/token
-H 'app-name:TheAppName'
Request: GET https://<domain-name>/wp-json/wp/v2/posts
Header: Authorization :Bearer <access_token /id_token>
Request: POST https://<domain-name>/wp-json/api/v1/token
Body:
grant_type = <client_credentials>
client_id = <client id>
client_secret = <client secret>
Sample curl Request Format-
curl -d "grant_type=client_credentials&client_id=<client_id>&client_secret=<client_secret>"
-X POST http://<wp_base_url>/wp-json/api/v1/token
-H 'app-name:TheAppName'
Request: POST https://<domain-name>/wp-json/api/v1/token
Body:
grant_type = <refresh_token>
refresh_token = <Refresh Token>
Sample curl Request Format-
curl -d "grant_type=refresh_token&refresh_token=<refresh_token>&client_id=<client_id>&client_secret=<client_secret>"
-X POST http://<wp_base_url>/wp-json/api/v1/token
-H 'app-name:TheAppName'
Request: GET https://<domain-name>/wp-json/wp/v2/posts
Header: Authorization : Bearer <access_token /id_token>
1. Refresh Token -
You can enable this option if you want to
receive the a string which is a refresh token as well along with access-token or JWT token in
the token endpoint request. It will allow the user to access the same resources as he was granted earlier. With the
new token created, user shuldn't get access beyond the original grant.
The refresh tokens allow authorization servers to use short time-periods (lifetime) for access tokens without
needing
to involve the user on token expiration.
With this token you can regenerate the access-token/JWT token as they are meant to expire shortly to increase
security.
2. Revoke Token -
Enabling this option allows you to revoke the existing access-token/JWT token to
make it invalid and hence the particular token cannot be used to authenticate the WP REST APIs. The RESTful API
validates the application credentials first and whether the token was issued to the application making the
revocation request.
In case the validation is not successful, the particular request is refused and error is shown in the application.
The API invalidates the token and the token cannot be used again after its revocation. Each revocation request
invalidates the tokens that were issued for the same type of
authorization grant.
var client = new RestClient("http://<wp_base_url>/wp-json/api/v1/token ");
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AlwaysMultipartFormDatatrue;
request.AddHeader("app-name", "TheAppName");
request.AddParameter("grant_type", "client_credentials");
request.AddParameter("client_id", "<client_id>");
request.AddParameter("client_secret", "<client_secret>");
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("grant_type", "client_credentials");
.addFormDataPart("client_id", "<client_id>");
.addFormDataPart("client_secret", "<client_secret>");
.build();
Request request = new Request.Builder()
.url("http://<wp_base_url>/wp-json/api/v1/token ")
.method("POST", null)
.addHeader("app-name", "TheAppName")
.build();
Response responseclient.newCall(request).execute();
var form = new FormData();
form.append("grant_type", "client_credentials");
form.append("client_id", "<client_id>");
form.append("client_secret", "<client_secret>");
var settings = {
"url": "http://<wp_base_url>/wp-json/api/v1/token ",
"method": "POST",
"timeout": 0,
"headers": {"app-name": "TheAppName"}
"processData": false,
"mimeType": "multipart/form-data",
"contentType": false,
"data": form
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array
(
CURLOPT_URL => 'http://%3Cwp_base_url%3E/wp-json/api/v1/token',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array( 'app-name: TheAppName' )
CURLOPT_POSTFIELDS => array('grant_type' => 'client_credentials','client_id' => '<client_id>','client_secret' => '<client_secret>'),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import mimetypes
from codecs import encode
conn = http.client.HTTPSConnection("<wp_base_url>")
dataList= []
boundary = 'wL36Yn8afVp8Ag7AmP8qZ0SA4n1v9T'
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=grant_type;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("client_credentials"))
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=client_id;'))
dataList.append('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<client_id>"))
dataList.append(encode('--'+ boundary))
dataList.append(encode('Content-Disposition: form-data; name=client_secret;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<client_secret<"))
dataList.append(encode('--'+boundary+'--'))
dataList.append(encode(''))
body = b'\r\n'.join(dataList)
payload= body
headers = {
'Content-type': 'multipart/form-data; boundary={}'.format(boundary),
'app-name': 'TheAppName'
}
conn.request("POST", "/wp-json/api/v1/token ", payload, headers)
res= conn.getresponse()
data = res.read()
print (data.decode("utf-8"))
var client = new RestClient("http://<wp_base_url>/wp-json/api/v1/token ");
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AlwaysMultipartFormData true;
request.AddHeader("app-name", "TheAppName");
request.AddParameter("grant_type", "password");
request.AddParameter("username", "<wordpress_username>");
request.AddParameter("password", "<wordpress_password>");
request.AddParameter("client_id", "<client_id>");
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("grant_type", "password");
.addFormDataPart("username", "<wordpress_username>");
.addFormDataPart("password", "<wordpress_password>");
.addFormDataPart("client_id", "<client_id>");
.build();
Request request = new Request.Builder()
.url("http://<wp_base_url>/wp-json/api/v1/token ")
.method("POST", null)
.addHeader("app-name", "TheAppName")
.build();
Response responseclient.newCall(request).execute();
var form = new FormData();
form.append("grant_type", "password");
form.append("username", "<wordpress_username>");
form.append("password", "<wordpress_password>");
form.append("client_id", "<client_id>");
var settings = {
"url": "http://<wp_base_url>/wp-json/api/v1/token ",
"method": "POST",
"timeout": 0,
"headers": {"app-name": "TheAppName"}
"processData": false,
"mimeType": "multipart/form-data",
"contentType": false,
"data": form
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array
( CURLOPT_URL => 'http://%3Cwp_base_url%3E/wp-json/api/v1/token%20',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array( 'app-name: TheAppName' )
CURLOPT_POSTFIELDS => array('username' => '<wordpress_username>','password' => '<wordpress_password>'),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import mimetypes
from codecs import encode
conn = http.client.HTTPSConnection("<wp_base_url>")
dataList= []
boundary = 'wL36Yn8afVp8Ag7AmP8qZ0SA4n1v9T'
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=grant_type;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("password"))
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=username;')
dataList.append('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<wordpress_username>"))
dataList.append(encode('--'+ boundary))
dataList.append(encode('Content-Disposition: form-data; name=password;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<wordpress_password<"))
dataList.append(encode('--'+boundary))
dataList.append(encode('Content-Disposition: form-data; name=client_id;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<client_id>"))
dataList.append(encode('--'+boundary+'--'))
dataList.append(encode(''))
body = b'\r\n'.join(dataList)
payload= body
headers = {
'Content-type': 'multipart/form-data; boundary={}'.format(boundary),
'app-name': 'TheAppName'
conn.request("POST", "/wp-json/api/v1/token ", payload, headers)
res= conn.getresponse()
data = res.read()
print (data.decode("utf-8"))
var client = new RestClient("http://<wp_base_url>/wp-json/api/v1/token ");
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AlwaysMultipartFormDatatrue;
request.AddHeader("app-name", "TheAppName");
request.AddParameter("grant_type", "refresh_token");
request.AddParameter("client_id", "<client_id>");
request.AddParameter("client_secret", "<client_secret>");
request.AddParameter("refresh_token", "<refresh_token>");
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("grant_type", "refresh_token");
.addFormDataPart("client_id", "<client_id>");
.addFormDataPart("client_secret", "<client_secret>");
.addFormDataPart("refresh_token", "<refresh_token>");
.build();
Request request = new Request.Builder()
.url("http://<wp_base_url>/wp-json/api/v1/token ")
.method("POST", null)
.addHeader("app-name", "TheAppName")
.build();
Response responseclient.newCall(request).execute();
var form = new FormData();
form.append("grant_type", "refresh_token");
form.append("client_id", "<client_id>");
form.append("client_secret", "<client_secret>");
form.append("refresh_token", "<refresh_token>");
var settings = {
"url": "http://<wp_base_url>/wp-json/api/v1/token ",
"method": "POST",
"timeout": 0,
"headers": {"app-name": "TheAppName"}
"processData": false,
"mimeType": "multipart/form-data",
"contentType": false,
"data": form
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array
(
CURLOPT_URL => 'http://%3Cwp_base_url%3E/wp-json/api/v1/token',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array( 'app-name: TheAppName' )
CURLOPT_POSTFIELDS => array('grant_type' => 'refresh_token','client_id' => '<client_id>','client_secret' => '<client_secret>','refresh_token' => '<refresh_token>'),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import mimetypes
from codecs import encode
conn = http.client.HTTPSConnection("<wp_base_url>")
dataList= []
boundary = 'wL36Yn8afVp8Ag7AmP8qZ0SA4n1v9T'
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=grant_type;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("refresh_token"))
dataList.append(encode('--' + boundary))
dataList.append(encode('Content-Disposition: form-data; name=client_id;'))
dataList.append('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<client_id>"))
dataList.append(encode('--'+ boundary))
dataList.append(encode('Content-Disposition: form-data; name=client_secret;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<client_id>"))
dataList.append(encode('--'+ boundary))
dataList.append(encode('Content-Disposition: form-data; name=refresh_token;'))
dataList.append(encode('Content-Type: {}'.format('text/plain')))
dataList.append(encode(''))
dataList.append(encode("<refresh_token<"))
dataList.append(encode('--'+boundary+'--'))
dataList.append(encode(''))
body = b'\r\n'.join(dataList)
payload= body
headers = {
'Content-type': 'multipart/form-data; boundary={}'.format(boundary),
'app-name': 'TheAppName'
conn.request("POST", "/wp-json/api/v1/token ", payload, headers)
res= conn.getresponse()
data = res.read()
print (data.decode("utf-8"))
var client = new RestClient("http://<wp_base_url>/wp-json/wp/v2/posts");
client.Timeout = -1;
var request = new RestRequest(Method.GET);
request.AddHeader = ("Authorization", "Bearer < access_token / id_token >");
request.AddHeader = ("app-name", "TheAppName");
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("http://<wp_base_url>//wp-json/wp/v2/posts")
.method("GET", body)
.addHeader = ("Authorization", "Bearer < access_token / id_token >");
.addHeader = ("app-name", "TheAppName");
.build();
Response responseclient.newCall(request).execute();
var settings = {
"url": "http://<wp_base_url>/wp-json/wp/v2/posts ",
"method": "GET",
"timeout": 0,
"headers": {
"Authorization": "Bearer < access_token / id_token >",
"app-name": "TheAppName"
},
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array
(
curl_setopt_array($curl, array(
CURLOPT_URL => 'http://%3Cwp_base_url%3E/wp-json/wp/v2/posts%20',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer < access_token / id_token >',
'app-name: TheAppName'
);
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("<wp_base_url>")
payload = "
headers = {
'Authorization': 'Bearer < access_token / id_token >',
'app-name': 'TheAppName',
}
conn.request("GET", "/wp-json/wp/v2/posts ", payload, headers)
res= conn.getresponse()
data = res.read()
print (data.decode("utf-8"))
a) OAuth 2.0 password Grant method:
b) OAuth 2.0 Client Credentials Grant Method:
c) REST API request to obtain the actual resource:
This feature allows restricting the REST API access based on the user roles. You can whitelist the roles for which you want to allow access to the requested resource for the REST APIs. So whenever a REST API request is made by a user, his role will be fetched and only allowed to access the resource if his role is whitelisted.
How to configure it?
Note: The Role based restriction feature is valid for Basic authentication (Username: password), JWT method, and OAuth 2.0 (Password grant).
This feature provides an option to choose a custom header rather than the default ‘Authorization’ header.
It will increase the security as you have the header named with your ‘custom name’, so if someone makes the REST API request with a header as ‘Authorization’ then he won’t be able to access the APIs.
How to configure it?
This feature allows you to whitelist your REST APIs so these can be accessed directly without any authentication. Hence all these whitelisted REST APIs are publicly available.
How to configure it?
This feature is applicable for JWT and OAuth 2.0 methods which uses time based tokens to authenticate the WordPress REST API endpoints. This feature allows you to set the custom expiry for the tokens such that the token will no longer be valid once the token expires.
How to configure it?
Mail us on apisupport@xecurify.com for quick guidance(via email/meeting) on your requirement and our team will help you to select the best suitable solution/plan as per your requirement.
Need Help? We are right here!
Thanks for your inquiry.
If you dont hear from us within 24 hours, please feel free to send a follow up email to info@xecurify.com
This privacy statement applies to miniorange websites describing how we handle the personal information. When you visit any website, it may store or retrieve the information on your browser, mostly in the form of the cookies. This information might be about you, your preferences or your device and is mostly used to make the site work as you expect it to. The information does not directly identify you, but it can give you a more personalized web experience. Click on the category headings to check how we handle the cookies. For the privacy statement of our solutions you can refer to the privacy policy.
Necessary cookies help make a website fully usable by enabling the basic functions like site navigation, logging in, filling forms, etc. The cookies used for the functionality do not store any personal identifiable information. However, some parts of the website will not work properly without the cookies.
These cookies only collect aggregated information about the traffic of the website including - visitors, sources, page clicks and views, etc. This allows us to know more about our most and least popular pages along with users' interaction on the actionable elements and hence letting us improve the performance of our website as well as our services.